如何使用 Google Analytics Data API
Google Analytics 是一個強大的網站分析工具,它可以讓您追蹤網站上的流量、轉換率和其他重要指標。
然而,對於那些想要分析 Google Analytics 數據的人來說,手動提取和處理這些數據非常浪費時間。這就是為什麼我們需要 Google Analytics Data API 的地方,可以讓你通過程式碼呼叫的方式從 Google Analytics 中取得數據,並將它們導入你的應用程式或進行進一步的數據分析。
在本文中,將介紹各位如何使用 Python 呼叫 Google Analytics Data API。
Google Analytics
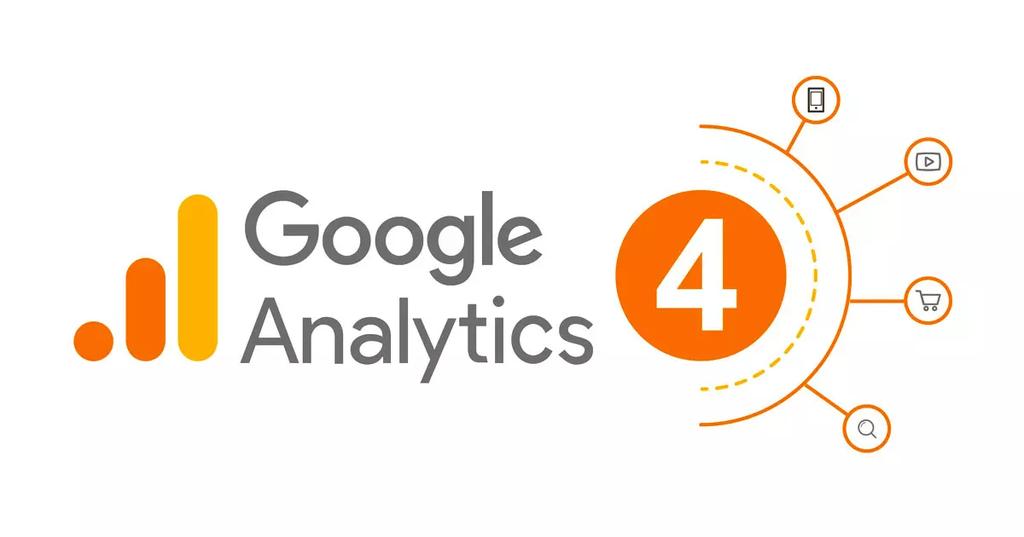
如果你還沒有接觸過 Google Analytics,那麼可以先參考 Google Analytics 的新手教學文件:
https://analytics.google.com/analytics/academy/course/6?hl=zh-tw
Google Analytics Data API
Google Analytics Data API 是一個 RESTful API,可以讓你從 Google Analytics 中取得數據。
設定 Google Cloud Platform
首先,我們需要在 Google Cloud Platform 專案裏面,啟用 Google Analytics Data API,並設定好服務帳戶的金鑰。
- 前往 Google Cloud Platform 控制台
- 建立一個新的專案,並輸入你想要的名字
- 啟用 Google Analytics Data API,選擇啟用 API 和服務
- 搜尋 Google Analytics Data API
- 啟用 Google Analytics Data API
- 點選建立服務帳戶,填好資料後選建立並繼續
- 選擇服務帳戶角色,選擇擁有者,完成
- 進入剛剛建立的服務帳戶,選擇金鑰,建立新的金鑰,選擇 JSON 格式
- 將金鑰放到專案目錄下
到這裡,Google Cloud Platform 的設定就完成了。
設定 Google Analytics
接下來,我們需要在 Google Analytics 中,把剛剛的服務帳戶設定到 Google Analytics 中。
以我的例子服務帳戶是這個
[email protected]
- 前往 Google Analytics 控制台
- 左下角的齒輪 管理 -> 帳戶存取管理
- 右上角選新增使用者
- 選擇分析人員
到這裡,Google Analytics 的設定就完成了。
呼叫 Google Analytics Data API
接下來,我們快要可以開始呼叫 Google Analytics Data API 了,現在還欠缺一個東西就是你想要查詢的 Google Analytics 的 view id。
取得 Google Analytics 的 view id
你可以在設定裡面找到你自己的 view id,下圖被遮起來的地方就是 view id。
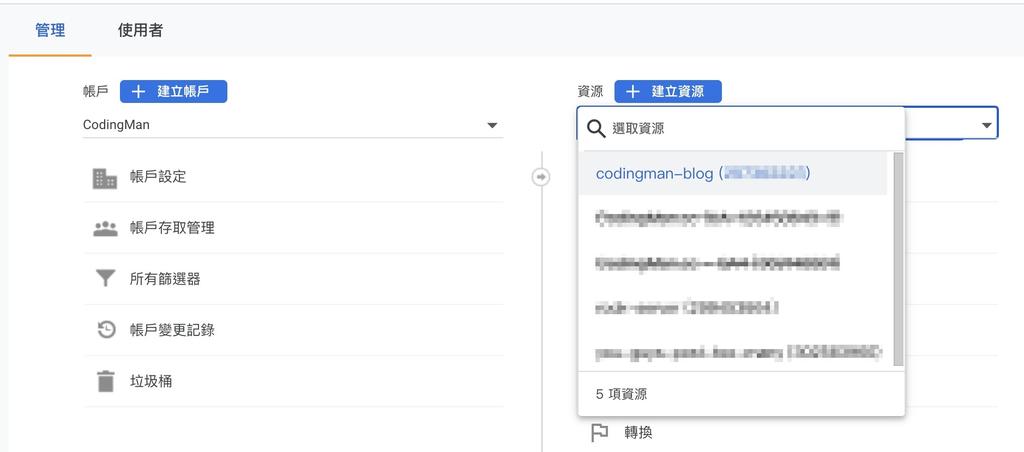
安裝 Google Analytics Data API SDK
接下來,我們需要安裝 Google Analytics Data API SDK,我們可以直接使用 pip 安裝。1
pip install --upgrade google-analytics-data
呼叫 Google Analytics Data API
終於,我們可以開始呼叫 Google Analytics Data API 了,我們直接來看程式碼。
這份程式碼是我用來更新熱門文章的,每天早上都會在 GitHub Actions 執行一次。
Workflow 可以參考
https://gist.github.com/PttCodingMan/e303178da7886baa6f2e08f2ae979658
其中比較需要注意的是需要把金鑰路徑設定到 GOOGLE_APPLICATION_CREDENTIALS
環境變數裏面。
為了安全,我也把 view id 一起放到環境變數裡面了。
至於 Google Analytics Data API 的一些變數欄位,可以參考官方文件:
https://developers.google.com/analytics/devguides/reporting/data/v11
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127import datetime
import os
import SingleLog
from SingleLog import Logger
from google.analytics.data_v1beta import RunReportRequest, BetaAnalyticsDataClient, Metric, \
DateRange, Dimension
# https://developers.google.com/analytics/devguides/reporting/data/v1
dimension_list = [Dimension(name="pageTitle"), Dimension(name="fullPageUrl")]
metrics_list = [Metric(name='screenPageViews')]
def query(logger: SingleLog.DefaultLogger, start_date: str, limit: int):
ignore_list = ['tags', 'undefined', 'archives', 'popular', 'about', 'side-projects']
report_request = RunReportRequest(
property=f'properties/{view_id}',
dimensions=dimension_list,
metrics=metrics_list,
limit=limit + 20,
date_ranges=[DateRange(start_date=start_date, end_date="today")],
)
report = BetaAnalyticsDataClient().run_report(report_request)
result = []
for row in report.rows:
title = row.dimension_values[0].value
if ' | CodingMan' in title:
title = title[:-12]
if not row.dimension_values[1].value.startswith('codingman.cc'):
logger.info('!! not codingman.cc: ', row.dimension_values[1].value)
continue
sub_url = row.dimension_values[1].value
sub_url = sub_url[12:]
if len(sub_url) == 1:
continue
ignore = False
for ig in ignore_list:
if f'/{ig}/' in sub_url:
ignore = True
break
if ignore:
continue
views = row.metric_values[0].value
logger.info('title', title)
logger.info('url', row.dimension_values[1].value, sub_url)
logger.info('views', views)
logger.info('-----------------')
result.append((title, sub_url, views))
if len(result) >= limit:
break
return result
if __name__ == '__main__':
logger = Logger('update_views')
if not os.path.exists(os.environ['GOOGLE_APPLICATION_CREDENTIALS']):
raise FileNotFoundError(f'{os.environ["GOOGLE_APPLICATION_CREDENTIALS"]} not found')
view_id = os.getenv('VIEW_ID')
with open('./source/popular/index.md', 'r') as f:
content = f.read()
# update recent days
date_range = 14
recent_days_reports = query(Logger(f'last_{date_range}_days'), f'{date_range}daysAgo', 5)
logger.info('full_history', len(recent_days_reports))
recent_days_content = '| 最新排名 | 文章 | 瀏覽量 |\n' \
'| -------- | -------- | -------- |\n'
rating = 1
for title, sub_url, views in recent_days_reports:
recent_days_content += f'| {rating} | [{title}]({sub_url}) | {views} |\n'
rating += 1
content = content.replace(
content[content.find('<!-- RECENT_DAYS:START -->'):content.rfind('<!-- RECENT_DAYS:END -->') + len(
'<!-- RECENT_DAYS:END -->')],
f'<!-- RECENT_DAYS:START -->\n{recent_days_content}<!-- RECENT_DAYS:END -->')
# update full history
full_history_reports = query(Logger('full_history'), '2021-12-14', 15)
logger.info('full_history', len(full_history_reports))
full_history_content = '| 最新排名 | 文章 | 瀏覽量 |\n' \
'| -------- | -------- | -------- |\n'
rating = 1
for title, sub_url, views in full_history_reports:
full_history_content += f'| {rating} | [{title}]({sub_url}) | {views} |\n'
rating += 1
content = content.replace(
content[
content.find('<!-- HISTORY:START -->'):content.rfind('<!-- HISTORY:END -->') + len('<!-- HISTORY:END -->')],
f'<!-- HISTORY:START -->\n{full_history_content}<!-- HISTORY:END -->')
# update update time
content = content.replace(
content[
content.find('<!-- UPDATE:START -->'):content.rfind('<!-- UPDATE:END -->') + len('<!-- UPDATE:END -->')],
f'<!-- UPDATE:START -->\nUpdate at {datetime.datetime.now().strftime("%Y.%m.%d")}\n<!-- UPDATE:END -->')
# write to file
with open('./source/popular/index.md', 'w') as f:
f.write(content)
結論
希望大家看完這篇文章之後,都可以順利使用 Google Analytics Data API。